반응형
자바 프로그램에서 DB와 관련된 작업을 처리할 수 있도록 도와주는 드라이버
Java DataBase Connectivity의 줄임말로, 자바에서 DB를 사용할 때 JDBC API를 이용하여 작업을 진행한다.
DB 종류 상관없이 하나의 JDBC를 사용하여 DB 작업을 처리한다.
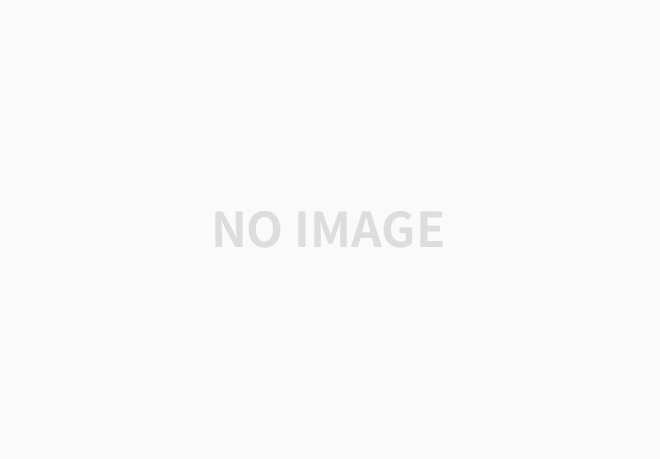
JDBC 드라이버는 각 DBMS에 알맞는 클라이언트를 의미한다. JDBC 프로그래밍 코딩 흐름을 요약하면 다음과 같다.
1. JDBC 드라이버 로드
2. DB 연결
- DriverManager.getConnection을 통해 데이터베이스 Connection을 구한다.
3. DB 작업(SQL Query)
- Query 작업을 위해 Statement 객체를 생성한다.
- Query를 실행한다.
- Query 실행 결과 사용
- Statement 종료
4. DB 연결 종료
JDBC 드라이버
- DBMS와 통신을 담당하는 자바 클래스
- DBMS 별로 알맞은 JDBC 드라이버 필요(jar)
- 로딩코드 : Class.forName("JDBC 드라이버 이름");
- MySQL : com.mysql.jdbc.Driver
- Oracle : oracle.jdbc.driver.OracleDriver
JDBC URL
- DBMS와의 연결을 위한 식별 값
- JDBC 드라이버에 따라 형식이 다름
- 구성 : jdbc:[DBMS]:[DB 식별자]
- MySQL : jdbc:mysql://HOST[:PORT]/DBNAME[?param=value¶m1=value2]
- Oracle : jdbc:oracle:thin:@HOST:PORT:SID
연결부분 예시 코드
/****************
- DriverManager를 이용해서 Connection 생성
- DriverManager.getConnection(String jdbcURL, String user, String password)
****************/
Connection conn = null;
try {
String jdbcDriver = "jdbc:mysql://localhost:3306/chap11?" + "useUnicode=true&characterEncoding=utf-8";
String dbUser = "jspexam";
String dbPass = "jspex";
conn = DriverManager.getConnection(jdbcDriver, dbUser, dbPass);
...
} catch(SQLException e){
//에러발생
} finally {
if (conn != null) try { conn.close(); } catch(SQLException e) {}
}
Statement를 이용한 쿼리 실행
/****************
- Connection.createStatement()로 Statement 생성
- Statement가 제공하는 메소드로 쿼리 실행
- SELECT : ResultSet executeQuery(String query)
- INSERT, UPDATE, DELETE : int executeUpdate(String query)
****************/
Statement stmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement();
int insertedCount = stmt.executeUpdate("insert...");
rs = stmt.executeQuery("select * from ...");
...
} catch(SQLException ex) {
...
} finally {
if (rs != null) try {rs. close(); } catch(SQLException ex) {}
if (stmt != null) try {stmt.close(); } catch(SQLException ex) {}
}
ResultSet에서 값 조회하기
- next() 메소드로 데이터 조회 여부 확인
- getString(), getInt(), getLong(), getFloat(), getDouble(), getTimestamp(), getDate(), getTime() 등등의 주요 메소드
Reference : https://dyjung.tistory.com/50
반응형
'개발 지식 > Java' 카테고리의 다른 글
[JAVA] Logback 사용법 (0) | 2020.02.19 |
---|---|
[Java] Generic 사용법 (0) | 2020.02.10 |
[JAVA] Servlet(서블릿) 이란 (0) | 2020.01.27 |
Mybatis Mapper 인터페이스 (0) | 2020.01.20 |
[Java] UnsatisfiedLinkError (0) | 2019.12.16 |